Build and push Docker images to AWS ECR using Github Actions
Table of Contents
Finding Docker as part of the deployment cycle when building modern applications is nothing new. This wide acceptance of Docker stems from the problem it solves—limited stress about the OS where your application will be deployed.
Docker containers provide a consistent environment, ensuring your application behaves the same way wherever it's run. But Docker on its own isn't enough. Once your application is containerized, you need a reliable way to store and manage these Docker images.
This is where Amazon Elastic Container Registry (ECR) comes into play. ECR provides a secure and highly available image registry that integrates with AWS services.
Before all this, you'd use a Version Control System (VCS) to upload the source code for your application, with GitHub being a popular option. But why use another tool to create your deployment pipeline when you can rely on an in-house solution—GitHub Actions?
Directly from GitHub, you can build and push your Docker image to AWS ECR with just a few lines of code. That's the exact goal of this tutorial.
In this article, we'll walk you through automating your deployment workflow using GitHub Actions to build and push Docker images to Amazon Elastic Container Registry (ECR). By the end of this tutorial, you'll have a more streamlined process for deploying your Docker images to AWS ECR.
Prerequisites
To properly follow through with this tutorial, you must have the following setup:
- AWS ECR repository: This is where your Docker images will live. Ensure you've created a repository in Amazon ECR, as we'll push our Docker image there.
- AWS IAM credentials: You'll need an IAM user with the appropriate permissions to access and push to your ECR repository. Specifically, you'll need your AWS Access Key ID and Secret Access Key. You can create these in the AWS console if you haven't set them up yet.
These two items are essential. Without them, you won’t be able to proceed with the automation process we’re about to set up.
Application setup
For this tutorial, we'll work with a simple word counter application built with JavaScript.
While this is the app we'll be using, you can actually apply the same steps to any application you choose—the focus here is on pushing the Docker image to AWS ECR, not the specific app itself.
To get the word counter application, you must fork and clone it from GitHub. The URL to the repository is below:
https://github.com/Aahil13/word-counter
After that, navigate to the folder and inspect the application’s source code and the Dockerfile.
To make it easier to follow along, you might want to delete the contents of the .github/workflows/docker-image.yml
file. This will help you build everything from scratch and get a better hands-on experience.
Encore is the Development Platform for building event-driven and distributed systems. Move faster with purpose-built local dev tools and DevOps automation for AWS/GCP. Get Started for FREE today.
Project Setup
In this section, we’ll walk through the essential steps to build and push our Docker image to AWS ECR using GitHub Actions. Here’s a quick rundown of what we’ll be doing:
- Set up secrets: First, we need to securely store our AWS Access Key ID and Secret Access Key in GitHub Secrets. This ensures that our workflow has the necessary permissions to interact with AWS ECR without exposing sensitive information.
- Create and write the workflow file: Next, we’ll create a GitHub Actions workflow file. This file will define the steps for building and pushing our Docker image to ECR. We’ll write the necessary YAML configuration to automate these tasks.
- Push to GitHub: Once our workflow file is set up, we’ll push all changes to our GitHub repository. This will trigger GitHub Actions to run the workflow.
- Confirm deployment: Finally, we’ll verify that our Docker image has been successfully pushed to AWS ECR. We’ll check the ECR console to ensure the image appears as expected and confirm that the deployment is complete.
Step 1: Setting up GitHub secrets
Copy your IAM credentials and store them in GitHub as secrets. This is important because, without them, GitHub Actions wouldn’t be able to authenticate and push your Docker image to your repository.
To add these secrets, head over to your GitHub repository and navigate to Settings > Secrets and variables > Actions.
Click on the New repository secret button and include the following credentials as secrets:
- AWS_ACCESS_KEY_ID: Enter your AWS access key ID.
- AWS_SECRET_ACCESS_KEY: Input your AWS secret access key.
- AWS_REGION: Specify the region where your ECR repository is located, such as
us-west-2
. - ECR_REPOSITORY: Provide the name of your ECR repository, for example,
word-counter
. If you'd prefer, you can also use the URL of the ECR repository.
Once done, you should have your secrets looking like this:
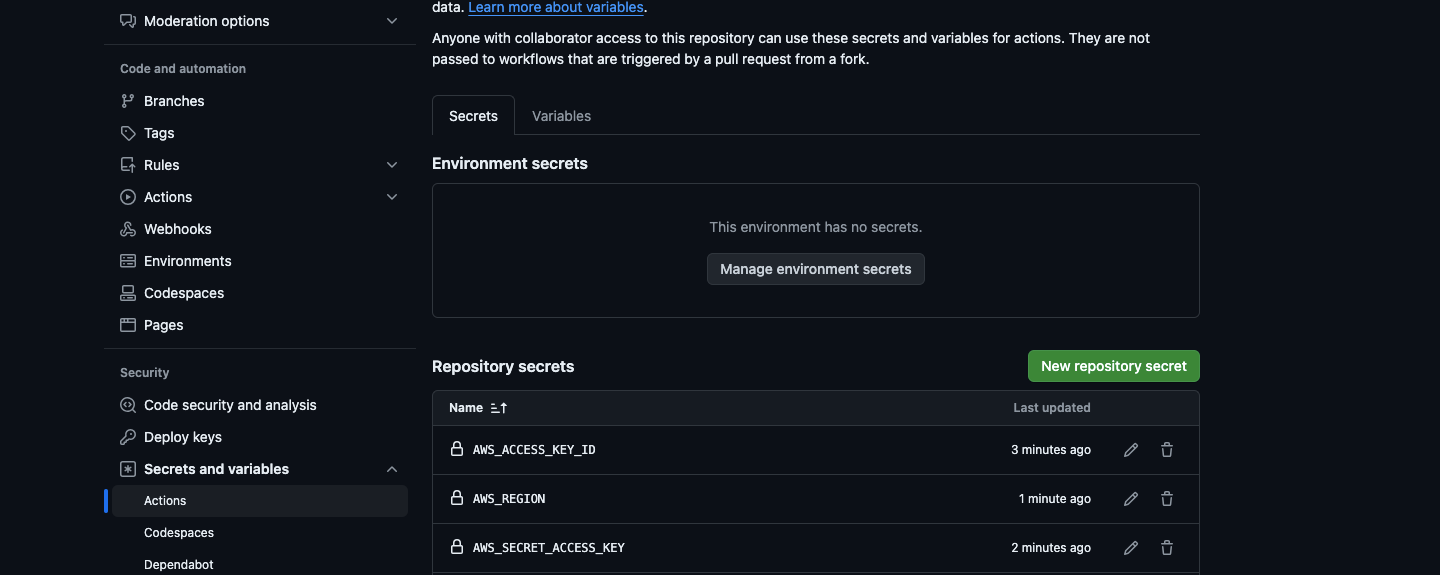
Figure 1: AWS ECR credentials added as GitHub secrets
Step 2: Create and write a GitHub workflow file
Now, you'll create a GitHub Actions workflow file that will automate the process of building and pushing your Docker image to ECR.
Create the workflow file
In your GitHub repository, navigate to the .github/workflows
directory. If it doesn't exist, create it.
Inside that directory, you can create the workflow file and name it whatever you want. In this case, it's called docker-image.yml
.
Add the following content
name: Build and Push Docker Image to AWS ECR
on:
push:
branches:
- main
jobs:
build-and-push:
runs-on: ubuntu-latest
steps:
- name: Checkout code
uses: actions/checkout@v3
- name: Configure AWS credentials
uses: aws-actions/configure-aws-credentials@v3
with:
aws-access-key-id: ${{ secrets.AWS_ACCESS_KEY_ID }}
aws-secret-access-key: ${{ secrets.AWS_SECRET_ACCESS_KEY }}
aws-region: ${{ secrets.AWS_REGION }}
- name: Log in to AWS ECR
run: |
aws ecr get-login-password --region ${{ secrets.AWS_REGION }} | docker login --username AWS --password-stdin ${{ secrets.ECR_REPOSITORY }}
- name: Build Docker image
run: |
docker build -t ${{ secrets.ECR_REPOSITORY }}:latest .
- name: Tag Docker image
run: |
docker tag ${{ secrets.ECR_REPOSITORY }}:latest ${{ secrets.ECR_REPOSITORY }}:latest
- name: Push Docker image to AWS ECR
run: |
docker push ${{ secrets.ECR_REPOSITORY }}:latest
Below is a brief explanation of what's going on in the code above:
- Checkout code: This step uses the
actions/checkout@v3
action to pull your code from the repository. - Configure AWS credentials: This step uses the
aws-actions/configure-aws-credentials@v3
action to set up the AWS CLI environment. It ensures that the AWS CLI has access to theAWS_ACCESS_KEY_ID
,AWS_SECRET_ACCESS_KEY
, andAWS_REGION
secrets that you configured in GitHub. - Log in to AWS ECR: This step logs in to your AWS ECR using the AWS CLI and your credentials.
- Build Docker image: This step builds the Docker image using the
Dockerfile
in your repository. - Tag Docker image: This step tags the Docker image with the
latest
tag. - Push Docker image to AWS ECR: This step pushes the tagged Docker image to your AWS ECR repository.
Step 3: Push changes to GitHub
Immediately after making your changes, you can commit and push them back to GitHub. This will trigger the GitHub Actions workflow to start building and pushing your Docker image to AWS ECR.
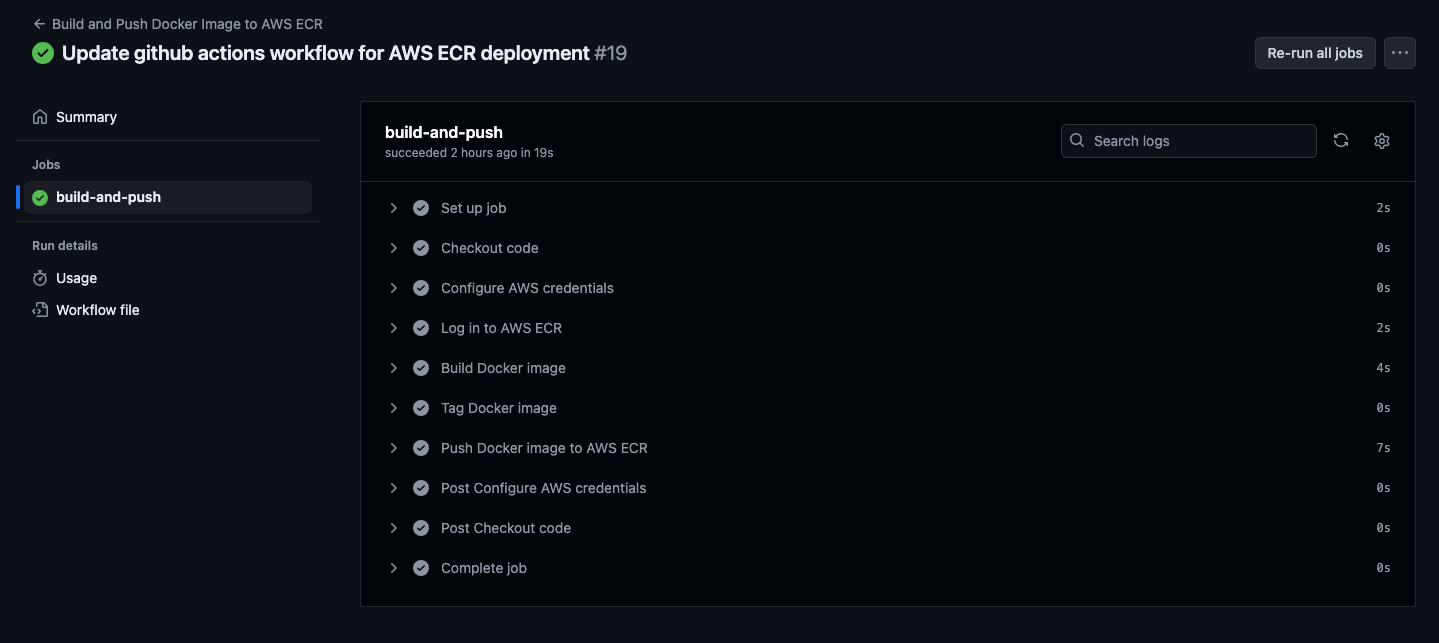
Figure 2: Successful output of your workflow file
Step 4: Verify the image in AWS ECR
If you followed this tutorial correctly, the output of running the workflow file should resemble the image above. From here, you can go back to your AWS Management Console.
Navigate to the Elastic Container Registry (ECR), select your repository and check if the Docker image has been pushed successfully.
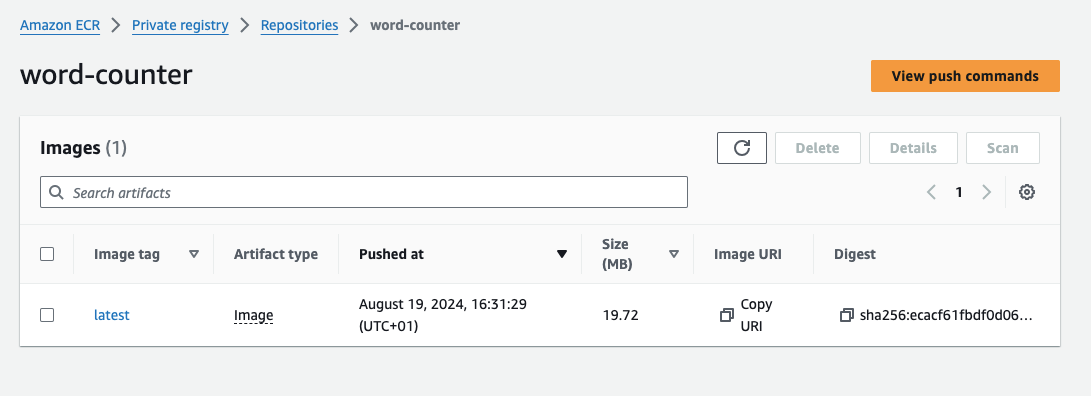
Figure 3: Docker image successfully deployed to AWS ECR
Encore is the Development Platform for building event-driven and distributed systems. Move faster with purpose-built local dev tools and DevOps automation for AWS/GCP. Get Started for FREE today.
Common issues
Even with everything set up correctly, you might still encounter a few hiccups when running this workflow.
Below are two common issues and how to resolve them:
Unable to locate credentials
This error typically occurs if the AWS credentials are not correctly set in GitHub Secrets or the secret names are typographically incorrect.
The error message looks something like this:
Unable to locate credentials. You can configure credentials by running "aws configure".
Error: Process completed with exit code 1.
To solve this issue, double-check that your secrets—AWS_ACCESS_KEY_ID
, AWS_SECRET_ACCESS_KEY
, and AWS_REGION
—are properly configured and correctly referenced in the workflow file. Also, ensure that your IAM user has the necessary permissions to interact with ECR.
Server misbehaving due to incorrect ECR repository name
This issue arises when there's a mistake in the name of your ECR repository. The Docker client is trying to access a non-existent repository, which leads to a DNS resolution error.
The error message looks like this:
Error response from daemon: Get "https://***/v2/": dial tcp: lookup *** on 127.0.0.53:53: server misbehaving
To fix this, double-check the ECR_REPOSITORY
secret and ensure it matches the exact name of your repository in AWS ECR. Once corrected, re-run the workflow, and the issue should be resolved.
Conclusion
This tutorial took you through the steps of building and pushing a Docker image to AWS ECR using GitHub Actions. You learned how to configure your GitHub secrets, create a workflow file, push your changes to GitHub, and verify that the image has been successfully pushed to AWS ECR.
By now, you should be feeling pumped. You can take this tutorial further by trying out this process on several projects. Make sure to switch up the workflow to suit your needs.
Like this article? Sign up for our newsletter below and become one of over 1000 subscribers who stay informed on the latest developments in the world of DevOps. Subscribe now!
The Practical DevOps Newsletter
Your weekly source of expert tips, real-world scenarios, and streamlined workflows!